How to Automate Clicking a Button on a Website with Selenium
Read on to find out how to setup your Python environment and use a script that demonstrates how to use Selenium with Python to automate button clicks on a website.
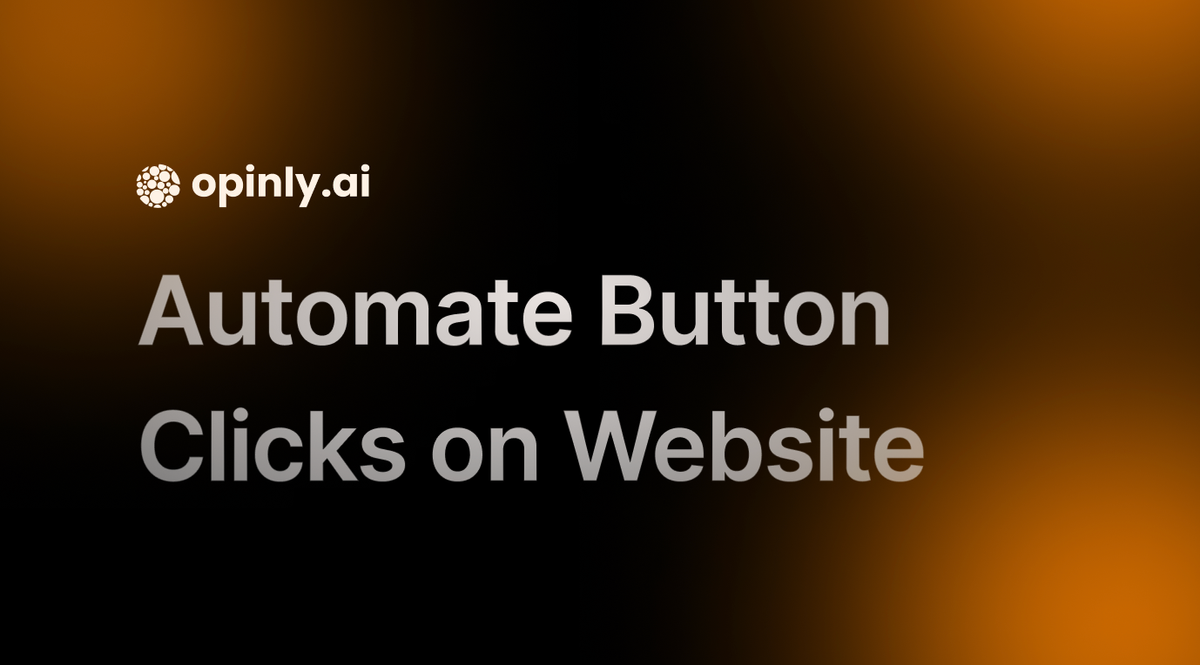
In this guide, we’ll explore how to automate the process of clicking buttons on a website using Selenium, a powerful tool for controlling web browsers through programs. We’ll write a simple Python script that automates button clicks, a technique useful for various tasks like testing websites. Setting up a bot to automate clicks on various buttons is straightforward and can be done with ease. Additionally, we will demonstrate how to use code snippets for automating button clicks, catering to both coding enthusiasts and those who prefer no-code solutions.
Introduction to Automating Repetitive Tasks
Automating repetitive tasks is a crucial aspect of increasing productivity and efficiency in various industries. Repetitive tasks, such as filling out web forms, extracting data, and clicking buttons, can be time-consuming and prone to errors. Automating these tasks can help reduce the workload, minimize errors, and free up time for more strategic and creative work. In this section, we will explore the benefits of automating repetitive tasks and introduce the concept of web page interactions.
Understanding Web Page Interactions
Web page interactions refer to the actions that a user performs on a web page, such as clicking buttons, filling out forms, and extracting data. Understanding web page interactions is essential for automating repetitive tasks. Web page interactions can be categorized into several types, including:
- Clicking buttons and links
- Filling out web forms
- Extracting data from web pages
- Navigating through web pages
Each type of web page interaction requires a different approach to automation. In the following sections, we will delve into the details of automating each type of web page interaction.
Setting Up Your Environment to Automate Running Selenium Scripts in Jupyter Notebook
To run Selenium scripts, particularly for automating button clicks on a website using a Jupyter Notebook, you need to set up your environment as follows:
Step 1: Install Python 3 and Jupyter Notebook:
- Download the latest Python 3 installer from the official website: https://www.python.org/downloads/ (choose the right version for your OS).
- Run the installer and ensure you add Python to your system path (recommended for easy access).
- Verify the installation by opening a terminal and typing python –version (or python3 –version on some systems).
Once Python 3 is installed, you can install Jupyter Notebook using pip:
- Open your terminal and run pip install notebook.
- Launch Jupyter Notebook by running jupyter notebook in your terminal.
Tip: Anaconda (a free Python distribution) also includes Jupyter Notebook: https://www.anaconda.com/blog/anaconda-individual-edition-2021-11
Step 2: Install Selenium
Selenium is a suite of tools for automating web browsers. Install it using Python’s package manager by running pip install selenium.
Step 3: Download WebDriver
For this example, we’re using Google Chrome as the browser. Therefore, you need to download ChromeDriver, which is a standalone server that implements WebDriver’s wire protocol for Chrome. Ensure the ChromeDriver version matches your Chrome browser’s version.
Step 4: Create a New Python Notebook
In the Jupyter Notebook interface, create a new Python notebook where you can write and execute your Selenium script. The following code snippet will be used in the script to automate interactions.
When selecting elements within a row or list in JavaScript, it is important to use the class name to identify and manipulate HTML elements effectively.
Writing the Script for Web Forms
Here’s a basic script outline:
Traditionally, coding has been the primary method for automating tasks within a web browser, such as clicking buttons and navigating websites.
Key Points of the Script
- Loop for Repeated Actions: We use a loop to repeat the process.
- Timing: time.sleep(3) sets a delay to prevent rapid-fire requests, which is essential for not overwhelming the server or getting flagged as spam.
- Incognito Mode: Running the browser in incognito mode helps to avoid issues with cached data and cookies.
- Finding Elements: driver.find_element is used to locate the button by its CSS selector. Ensure you have the correct selector for the button you intend to click.
- Error Handling: The try-except block is crucial for handling exceptions and avoiding crashes.
- Recording Key Presses: Recording key presses can enhance the functionality of your automation script, allowing for more complex interactions and better control over the web application.
- Inspecting Selected Elements: Inspecting selected elements is important for tasks like data extraction and automation processes. Visual cues, such as colors representing different statuses, can help identify the elements chosen for specific actions.
Selecting Elements for the Click Action
Selecting elements for the click action is a critical step in automating web page interactions. Elements can be selected using various methods, including:
- Using the Chrome extension to select elements
- Using the Eyedropper icon to select elements
- Using the aaname property to select elements
The method used to select elements depends on the complexity of the web page and the type of element being selected. In general, it is recommended to use the Chrome extension or the Eyedropper icon to select elements, as these methods are more accurate and efficient.
Automating Clicking on Multiple Buttons
Automating clicking on multiple buttons is a common requirement in web page interactions. This can be achieved using various methods, including:
- Using the Repeat click feature in Automatio
- Using the “For Each Row” activity to loop through a list of buttons
- Using JavaScript to automate clicking on multiple buttons
The method used to automate clicking on multiple buttons depends on the complexity of the web page and the type of buttons being clicked. In general, it is recommended to use the Repeat click feature or the “For Each Row” activity, as these methods are more efficient and accurate.
I hope this helps! Let me know if you need any further assistance.
Ethical Considerations and Best Practices for Automating
Automating interactions with websites should be done responsibly, utilizing automated tools to perform tasks without manual intervention, such as logging into Gmail, extracting data from web pages, and clicking buttons on websites. Abide by the website’s terms of service and ensure your actions do not harm the website’s functionality or the user experience for others.
Conclusion
This script demonstrates a basic yet powerful way to automate browser tasks using Selenium in Python. It can be extended and modified for more complex scenarios and different types of web interactions. Always remember to use such automation responsibly and ethically.