On-Page SEO in React Native
Learn how to optimize your React Native website for search engines through server-side rendering, technical SEO implementation, and performance optimization. Overcome common challenges and improve your site's visibility.
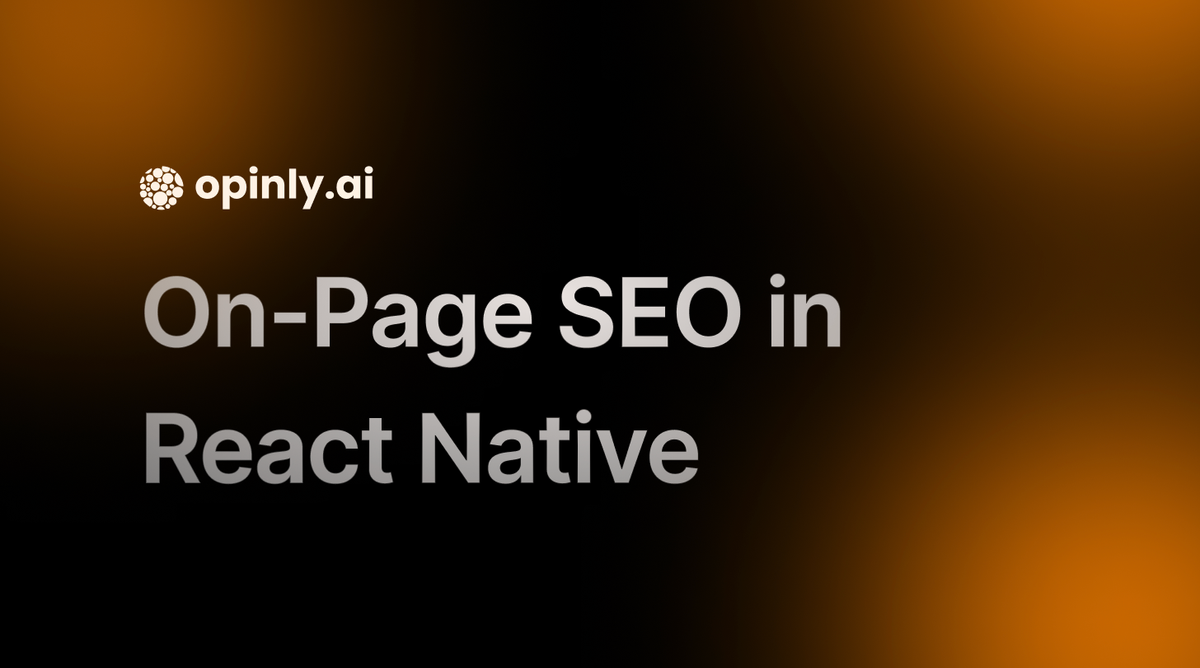
Introduction
React Native, primarily known for mobile app development, can present unique challenges when used for web development - particularly when it comes to Search Engine Optimization (SEO). This guide will help you navigate these challenges and implement effective SEO strategies for your React Native web projects.
Understanding the Challenge
React Native was originally designed for mobile application development, which means implementing traditional web SEO practices can be tricky. The main challenges include:
- Client-side rendering by default
- Limited access to HTML meta tags
- Dynamic content handling
- Slower initial page loads
- Search engine crawling difficulties
Best Solutions for React Native SEO
1. Server-Side Rendering (SSR)
The most effective solution for React Native web SEO is implementing Server-Side Rendering. Here's why and how:
- Benefits:
- Improved initial page load times
- Better search engine crawling
- Enhanced social media sharing
- Improved SEO performance
- Implementation Options:
- Next.js integration
- Gatsby for static site generation
- Custom SSR setup
2. Technical SEO Implementation
Meta Tags and Headers
import { Helmet } from 'react-helmet';
function YourComponent() {
return (
<>
<Helmet>
<title>Your Page Title</title>
<meta name="description" content="Your page description" />
<meta name="keywords" content="relevant, keywords, here" />
</Helmet>
// Your component content
</>
);
}
URL Structure
- Implement clean, SEO-friendly URLs
- Use proper routing with react-router
- Ensure proper handling of dynamic routes
3. Content Optimization
Best Practices:
- Use semantic HTML elements
- Implement proper heading hierarchy (H1, H2, H3)
- Optimize image alt tags
- Include relevant keywords naturally
- Create mobile-responsive layouts
4. Performance Optimization
Key Areas:
- Minimize bundle size
- Implement code splitting
- Optimize images and media
- Use lazy loading for components
- Implement caching strategies
Alternative Approaches
1. Hybrid Solution
Consider creating a parallel web version optimized for SEO while maintaining your React Native app for mobile users.
2. Static Site Generation (SSG)
For content-heavy sites, consider using static site generation tools like:
- Gatsby
- Next.js static generation
- React Static
Step-by-Step Implementation Guide
- Choose Your Rendering Strategy
- Evaluate SSR vs. SSG based on your needs
- Select appropriate framework (Next.js recommended)
- Set Up Basic SEO Elements
- Configure meta tags
- Implement robots.txt
- Create sitemap.xml
- Optimize Content Structure
- Implement proper heading hierarchy
- Structure content for featured snippets
- Add schema markup where applicable
- Monitor and Adjust
- Set up Google Search Console
- Monitor core web vitals
- Track ranking performance
- Make data-driven adjustments
Tools and Resources
SEO Tools:
- Google Search Console
- Google Analytics
- SEMrush or Ahrefs
- Lighthouse for performance metrics
Development Resources:
- Next.js documentation
- React Native Web documentation
- Google's SEO starter guide
Conclusion
While React Native presents unique challenges for web SEO, implementing the right strategies can help achieve good search engine visibility. Focus on server-side rendering, proper technical implementation, and continuous optimization to achieve the best results.
Remember that SEO is an ongoing process, not a one-time setup. Regular monitoring and adjustments based on performance data will help maintain and improve your site's search engine rankings.